Below is how do I search Microsoft 365 content programmatically from PowerShell using MS Graph API, PowerShell PnP, Microsoft Graph module, MSAL library being authenticated as user or daemon application. Let me focus on SharePoint content here but you can use the same technique to search through other Microsoft 365 services. Also, I’ll be using PowerShell but same ideas should work for other platforms/languages – Python, C#, node.js etc.
First, we need to be authenticated
Here is how to authenticate to Microsoft 365 Graph API.
Second, we need to be authorized
To search on behalf of currently authenticated user we need delegated “Sites.Read.All” API permissions. I recommend you to add both Graph API and SharePoint API permissions as different libraries might use different API’s under the hood. Ensure you add delegated “Sites.Read.All” even if you already have “Sites.FullControl.All” as by some reason “Sites.FullControl.All” does not always work for search.
Here is how an app API permissions to search as current user should look like:
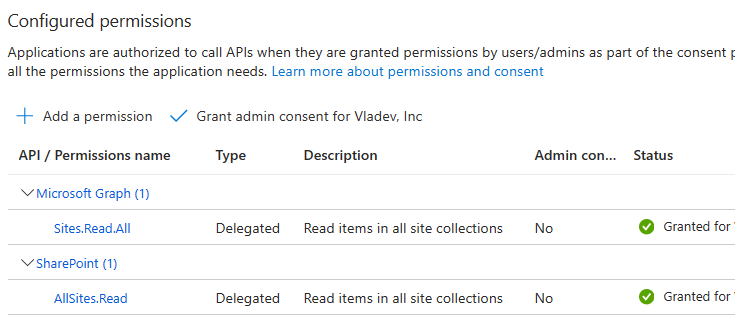
Actually, for interactive authentication app ownership is not required, so we can surely use our own registered app, but also it is possible to use any other app registered in Azure and properly configured, e.g. Enterprise “PnP Management Shell” app id: “31359c7f-bd7e-475c-86db-fdb8c937548e”
How do I create and configure Azure App with delegated permissions to SharePoint for PowerShel usage
For unattended search – e.g. search on behalf of daemon app – we need application “Sites.Read.All” API permissions. Again, I suggest both Graph API and SharePoint API permissions added. Here is how an app API permissions to search as daemon app should look like:
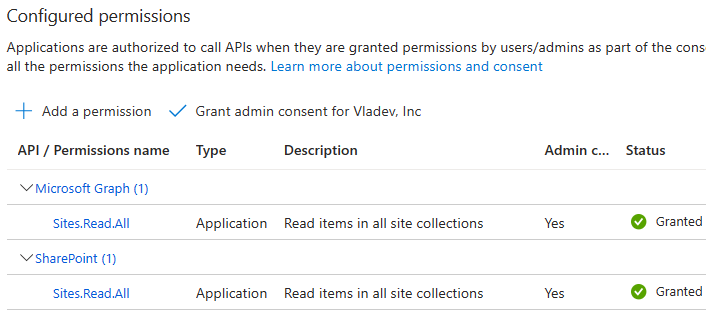
Ensure you got admin consent for API permissions.
In case you have incorrect permissions in your app – Microsoft Graph will be kind enough to inform you exactly what you need. Example:
“Access to ChatMessage in Graph API requires the following permissions: Chat.Read or Chat.ReadWrite, ChannelMessage.Read.All. However, the application only has the following permissions granted: Sites.Read.All, User.Read”
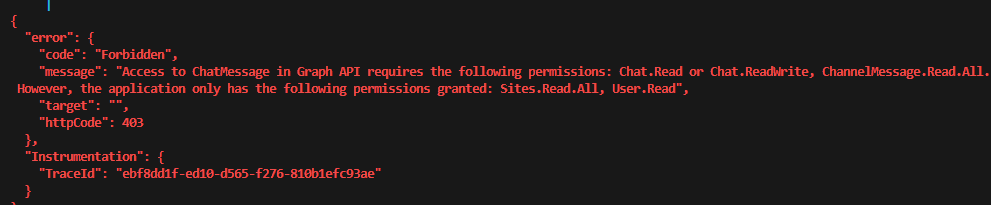
Assuming we have configured apps – let us get started with
Microsoft Graph API
Microsoft Graph API allows search through all the Microsoft 365 content – including Exchange e-mail messages, Yammer (Viva Engage) and Teams chat messages and surely OneDrive and SharePoint content (please refer to the original doc).
Authenticate as current user to Search with Graph API
I use MSAL.PS PowerShell module to get token, then I build a headers variable
# Prerequisites
Get-Module MSAL.PS -ListAvailable | ft name, Version, Path
# Install-Module MSAL.PS -Force -Scope CurrentUser -AcceptLicense
Import-Module MSAL.PS
# Interactive Authentication
$clientid = 'd82858e0-ed99-424f-a00f-cef64125e49c'
$TenantId = '7ddc7314-9f01-45d5-b012-71665bb1c544'
$token = Get-MsalToken -TenantId $TenantId -ClientId $clientid -Interactive
$headers = @{Authorization = "Bearer $($token.AccessToken)" }
Authenticate as service/daemon app
You’d need to update the script providing Tenant id, client (app) id and client (app) secret:
# App Authentication
$clientID = ""
$clientSc = ""
$TenantId = ""
# Construct URI and body needed for authentication
$uri = "https://login.microsoftonline.com/$tenantId/oauth2/v2.0/token"
$body = @{
client_id = $clientID
client_secret = $clientSc
scope = "https://graph.microsoft.com/.default"
grant_type = "client_credentials"
}
# Get OAuth 2.0 Token
$tokenRequest = Invoke-WebRequest -Method Post -Uri $uri -ContentType "application/x-www-form-urlencoded" -Body $body -UseBasicParsing
$token = ($tokenRequest.Content | ConvertFrom-Json).access_token
$headers = @{Authorization = "Bearer $token" }
$headers
Search m365 SharePoint and OD content with Microsoft Graph API
In this sample I limited search scope to list items only ($entityTypes = “[‘listItem’]”). Check other entity types here.
Code:
# Search
$entityTypes = "['listItem']"
$apiUrl = "https://graph.microsoft.com/beta/search/query"
$query = "*"
# body for interactive search
$body = @"
{
"requests": [
{
"entityTypes": $entityTypes,
"query": {
"queryString": "$query"
}
}
]
}
"@
$res = Invoke-RestMethod -Headers $Headers -Uri $apiUrl -Body $Body -Method Post -ContentType 'application/json'
$res.value[0].hitsContainers[0].hits
If you are getting error message “SearchRequest Invalid (Region is required when request with application permission.)”:
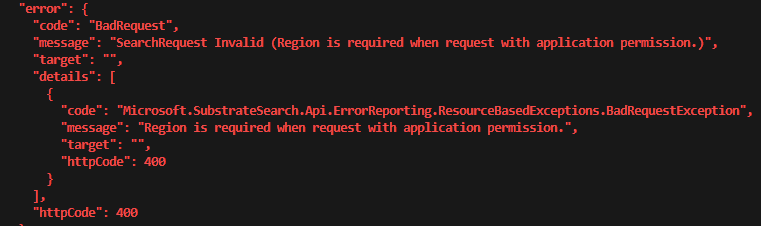
that’s OK, just modify your body to include region like this (“region”: “NAM” for North America or “GBR” or …). Also, I can modify body with from/size for paging (technique used to iterate through search results if there are many) and return just specific fields to decrease traffic and improve performance:
# Search
$entityTypes = "['driveItem','listItem','list','drive','site']"
$entityTypes = "['driveItem','listItem']"
$query = "LastModifiedTimeForRetention<2021-01-01"
$apiUrl = "https://graph.microsoft.com/beta/search/query"
$query = "test*"
$body = @"
{
"requests": [
{
"entityTypes": $entityTypes,
"query": {
"queryString": "$query"
},
"from" : 0,
"size" : 5,
"fields": ["WebUrl","lastModifiedBy","name" ],
"region": "NAM"
}
]
}
"@
$res = Invoke-RestMethod -Headers $Headers -Uri $apiUrl -Body $Body -Method Post -ContentType 'application/json'
$res.value[0].searchTerms
$res.value[0].hitsContainers[0].hits
$res.value[0].hitsContainers[0].hits.Count
We’d not use region for interactive calls or we’ll get “Region is not supported when request with delegated permission.”.
Microsoft.Graph PowerShell module
There is a Microsoft.Graph PowerShell module provided by Microsoft which simplifies authentication and search operations.
Interactive authentication code sample:
# Prerequisites
Get-Module Microsoft.Graph.Authentication -ListAvailable
Get-Module Microsoft.Graph.Search -ListAvailable
# Interactive Authentication
$clientid = '31359c7f-bd7e-475c-86db-fdb8c937548e'
$clientid = 'd82858e0-ed99-424f-a00f-cef64125e49c'
$TenantId = '7ddc7314-9f01-45d5-b012-71665bb1c544'
Connect-MgGraph -ClientId $clientid -TenantId $TenantId
For daemon app authentication we need a certificate configured in Azure App and installed on the user machine. Daemon app authentication code sample (please specify your tenant id, app (client) id and certificate thumbprint:
# App Authentication
$clientID = ""
$certThumbprint = ""
$TenantId = ""
Connect-MgGraph -ClientId $clientid -TenantId $TenantId -CertificateThumbprint $certThumbprint
Code sample for SharePoint search with Microsoft.Graph PowerShell module
As currently authenticated user
# Search in the current user context
$params = @{
requests = @(
@{
entityTypes = @(
"driveItem"
)
query = @{
queryString = "lorem"
}
from = 0
size = 25
fields = @(
"title"
"description"
)
}
)
}
$res = Invoke-MgQuerySearch -Body $params
$res.HitsContainers[0].Hits
Again, in case with app authentication – an additional parameter – region – is required:
# Search
$params = @{
requests = @(
@{
entityTypes = @(
"driveItem"
)
query = @{
queryString = "lorem"
}
from = 0
size = 25
fields = @(
"title"
"description"
)
region = "NAM"
}
)
}
$res = Invoke-MgQuerySearch -Body $params
$res.HitsContainers[0].Hits
It’s a good idea to explore returning object.
PnP.PowerShell module
PnP.PowerShell allows you to search through Microsoft 365 SharePoint content with PowerShell style – using command and options.
Let us authenticate interactively:
# Interactive Authentication
$clientid = 'd82858e0-ed99-424f-a00f-cef64125e49c'
$TenantId = '7ddc7314-9f01-45d5-b012-71665bb1c544'
$siteUrl = "https://s5dz3.sharepoint.com"
Connect-PnPOnline -ClientId $clientid -Tenant $TenantId -Url $siteUrl -Interactive
Authentication on behalf of service/daemon app would require certificate installed on the machine and configured in the app and look like:
# Application (daemon) Authentication
$clientID = ""
$certThumbprint = ""
$TenantId = ""
$siteUrl = "https://contoso.sharepoint.com"
Connect-PnPOnline -ClientId $clientid -Tenant $TenantId -Url $siteUrl -Thumbprint $certThumbprint
There are no differences in Microsoft 365 SharePoint Search with PowerShell code samples for interactive and daemon apps (no region parameter).
Examples:
# search
$query = "test*"
$res = Submit-PnPSearchQuery -Query $query
# examples of query:
$query = "test*"
$query = "* contentclass:STS_ListItem_DocumentLibrary"
$query = "* author:Patti"
$query = "test* site:https://s5dz3.sharepoint.com/teams/sxc"
# examples of submitting request
$res = Submit-PnPSearchQuery -Query $query
Submit-PnPSearchQuery -Query $query -All
Submit-PnPSearchQuery -Query $query -MaxResults 5
Submit-PnPSearchQuery -Query $query -SortList @{"LastModifiedTime" = "ascending"}
# exploring result object:
$res.ResultRows.Count
$res.ResultRows[0]
$res.ResultRows.Title
$res.ResultRows.OriginalPath
$res.ResultRows.LastModifiedTime
Video tutorials
References
- Search m365 SharePoint and Teams content programmatically via MS Graph API: index
- Microsoft Identity Platform
- App authentication to SharePoint via MS Graph API and SharePoint API
- Authenticate to SharePoint and MS Graph Interactively with Client App and MSAL token
- Use the Microsoft Search API to search OneDrive and SharePoint content
- Microsoft.Graph PowerShell module
- My GitHub repo with Microsoft 365 Search code samples
- PnP Submit-PnPSearchQuery